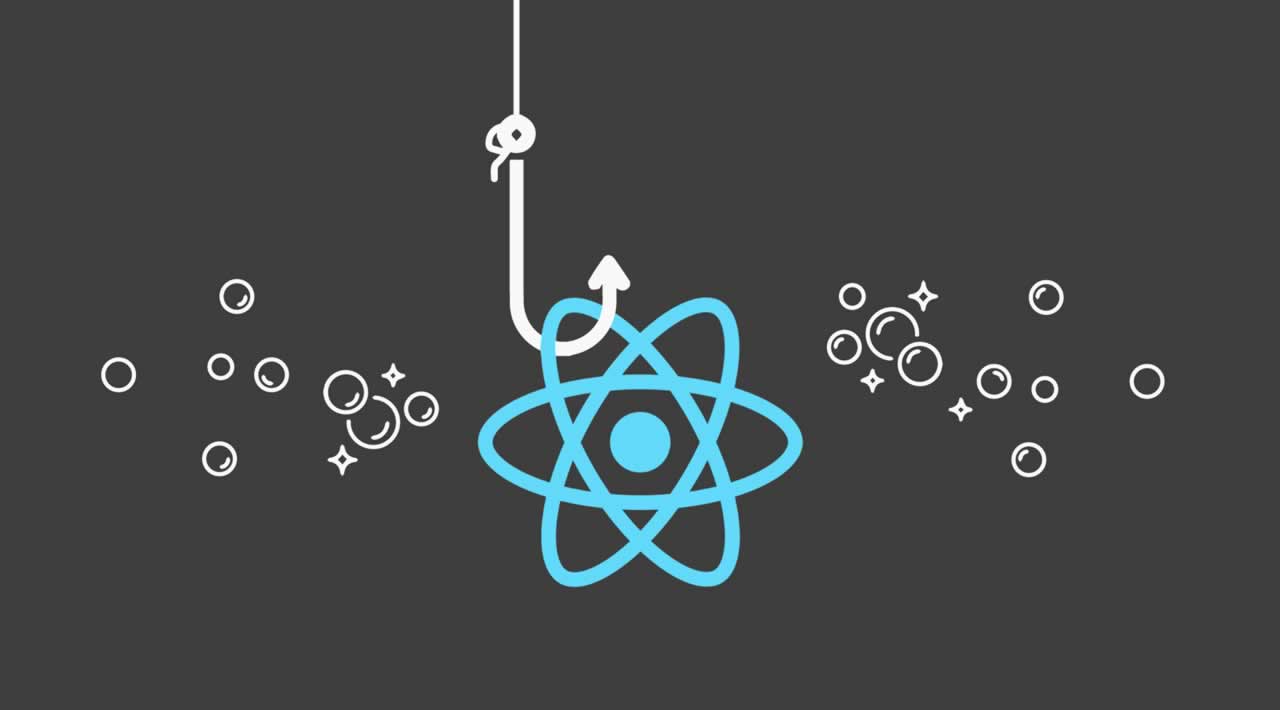
How to create your own custom React Hooks
React Hooks Definition
Hooks are a way to separate logic and share it across multiple components. Hooks provide a way to have repeatable and reusable logic for components, which helps to make components easier to maintain. Hooks are also easier to read and understand than class-based components, making them more accessible and easier to debug. Hooks are functions that let you “hook into” React state and lifecycle features from function components. Hooks don’t work inside classes.
The Advantages of Creating Custom React Hooks
You may reuse code more effectively, write less code, and make your code easier to read by using personalized React Hooks. You may write components more effectively and prevent code duplication by developing custom Hooks.
Writing a Custom React Hook
There are some guidelines that must be followed in order to create a custom React Hook:
-
The word “use” must be first in the name of the hook function. A unique hook might be called useLocalStorage or useAuthentication, for instance.
-
Hooks can only be invoked from other Hooks or at the top level of a functional component. This implies that a nested function or conditional expression cannot call a Hook.
-
Hooks should not be called conditionally.
-
Steer clear of putting hooks inside loops or conditions because doing so can result in unpredictable behavior.
-
Keep your hooks compact and concentrated on a single functional element. If a Hook is performing too many tasks, you might want to divide it into several Hooks.
Example of building react custom hook:
Here is an example of a custom React Hook for toggling dark mode called useDarkMode:
import { useState, useEffect } from 'react';
const useDarkMode = () => {
const [isDarkMode, setIsDarkMode] = useState(false);
useEffect(() => {
const body = document.body;
if (isDarkMode) {
body.classList.add('dark-mode');
} else {
body.classList.remove('dark-mode');
}
}, [isDarkMode]);
const toggleDarkMode = () => {
setIsDarkMode(!isDarkMode);
};
return [isDarkMode, toggleDarkMode];
};
export default useDarkMode;
In order to monitor whether dark mode is on or off, this Hook uses useState. The DOM is updated when the mode is toggled using useEffect as well. The Hook returns an array that contains two elements: the mode’s current status (isDarkMode), and a function to switch the mode on or off (toggleDarkMode).
You can use this Hook in your React components like this:
import useDarkMode from './useDarkMode';
function App() {
const [isDarkMode, toggleDarkMode] = useDarkMode();
return (
<div className="App">
<button onClick={toggleDarkMode}>
{isDarkMode ? 'Disable Dark Mode' : 'Enable Dark Mode'}
</button>
</div>
);
}
This example shows a simple button that toggles the dark mode on and off. When the button is clicked, the toggleDarkMode function is called, which updates the isDarkMode state and causes the DOM to be updated with the new mode.
NOTE: More example can be found on usehooks.com which is a website that provides a collection of custom React Hooks that you can use in your projects.
Conclusion
Finally, by allowing you to extract and reuse stateful logic between components, custom React Hooks can greatly simplify and modularize your code. By sticking to the Hooks rules, you can ensure that your Hooks are efficient, simple to use, and adhere to React best practices. When writing a custom Hook, it’s critical to identify the state and behavior you want to manage, set up state variables, and return an array of state and any state update functions. With these principles in mind, you can create powerful, reusable Hooks that improve the flexibility and maintainability of your code.